How to use React Bootstrap Table on React.js
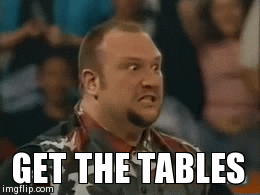
When it comes to building out an app that provides a user friendly table to display and manipulate data you want to make sure you use React Bootstrap Table. React Bootstrap Table allows you to add, delete, and edit all in one single area with out having to build out long prices of code. I’ll be walking you step by step to build you that best fits your app.
Step 1: Install React Bootstrap
You want to go into your vscode terminal and copy and paste the code below.
npm install react-bootstrap-table — save
Step 2: Import react-bootstrap-table in module
let ReactBsTable = require('react-bootstrap-table');let BootstrapTable = ReactBsTable.BootstrapTable;let TableHeaderColumn = ReactBsTable.TableHeaderColumn;
Step 3 Add CSS
import 'react-bootstrap-table/css/react-bootstrap-table.css';
Step 4 Create a react-bootstrap-table in your app
import React from 'react';import 'react-bootstrap-table/css/react-bootstrap-table.css';let ReactBsTable = require('react-bootstrap-table');let BootstrapTable = ReactBsTable.BootstrapTable;let TableHeaderColumn = ReactBsTable.TableHeaderColumn;class Task extends React.Component {
render() {let products = [{
id: 1,
name: "Product1",
price: 120 },
{id: 2,
name: "Product2",
price: 80 }...];return (<div className="task-container"><BootstrapTable data={products} cellEdit={ cellEditProp } striped hover ><TableHeaderColumn isKey={true} dataField='id' dataAlign='left' width='0' >Id</TableHeaderColumn><TableHeaderColumn dataField='name' dataAlign='left' width='40' >Task</TableHeaderColumn><TableHeaderColumn dataField='price' dataSort={true} headerAlign='right' dataAlign='right' width='80' >Comment</TableHeaderColumn></BootstrapTable></div>)}}export default Task
Step 5: Manipulation Delete and Create

I love react bootstrap table because of all the manipulation you can do with the table. One of the things you can do is delete an item with a click of a button. Let me show you how its done!
class Task extends React.Component {onDeleteRow = (e) => {this.props.onDeleteRow(e[0])}handleClick = (value) => {this.props.handleEdit(value)}render() {let itemDatas = this.props.tasks !== undefined ? this.props.tasks.map(task => task) : nullconst cellEditProp = {mode: 'click',afterSaveCell: this.handleClick}return (<div className="task-container"><BootstrapTable data={itemDatas} cellEdit={ cellEditProp } deleteRow={ true } selectRow={ { mode: 'radio' } } options={ { onDeleteRow: this.onDeleteRow } } striped hover ><TableHeaderColumn isKey={true} dataField='id' dataAlign='left' width='0' >Id</TableHeaderColumn><TableHeaderColumn dataField='name' dataAlign='left' width='40' >Task</TableHeaderColumn><TableHeaderColumn dataField='comment' dataSort={true} headerAlign='right' dataAlign='right' width='80' >Comment</TableHeaderColumn></BootstrapTable></div>)}}export default Task

function onAfterInsertRow(row) { let newRowStr = ''; for (const prop in row) {
newRowStr += prop + ': ' + row[prop] + ' \n';
} alert('The new row is:\n ' + newRowStr);
} const options = {
afterInsertRow: onAfterInsertRow // A hook for after insert rows
}; class InsertRowTable extends React.Component { render() { return ( <BootstrapTable data={ products } insertRow={ true } options={ options }>
<TableHeaderColumn dataField='id' isKey>Product ID</TableHeaderColumn>
<TableHeaderColumn dataField='name'>Product Name</TableHeaderColumn>
<TableHeaderColumn dataField='price'>Product Price</TableHeaderColumn>
</BootstrapTable>
);
}
}
Congratulations you now have a full interactive table to show your users!

React Bootstrap Table has way more to offer and I have put a link below so you can explore all the build in functions. Thanks for reading and don’t forget to like and share.