Using Chart.js to build animated graphs in JavaScript

Chart.js is a library that provides many type of chart like line, pie, or bar charts to display information. I’ll be taking you on a step by step process on installing and helping you get started on your next project.
- Installing Chart.js in Javascript
npm install chart.js --save
2. Create a canvas in your HTML file
<canvas id="pieChart"></canvas>
3. Include Chart.js in our HTML page with a script tag at the top.
<script src="https://cdn.jsdelivr.net/npm/chart.js@2.8.0"></script>
4. Create an index.js file to put all this code in.
let pieChart = document.getElementById('my-pie-chart').getContext('2d');let pieChartSize = document.getElementById('my-pie-chart')pieChartSize.style.height = '128px'let newPie = new Chart(pieChart, {// The type of chart we want to createtype: 'pie',// The data for our datasetdata: {labels: ['Bills', 'Entertainment', 'Rent'],datasets: [{label: 'My First dataset',backgroundColor: ['rgb(252, 223, 0)','rgb(255, 166, 0)','rgb(255, 0, 201)'],borderColor: ['rgb(252, 223, 0)','rgb(255, 166, 0)','rgb(255, 0, 201)'],data: [30, 10, 20]}]},// Configuration options go hereoptions: {layout: {padding: {left: 50,right: 0,top: 0,bottom: 0}}}});
5. Now you want to call this index.js file on your html with a script tag under your chart.js scrip tag.
<script defer src="src/index.js"></script>
6. Now you should be able to go on your terminal an open index.html and see your pie chart.

Great job you created a pie chart in Javascript!

Now let’s mix it up a bit and change the type of graph we have. On your index.js file go ahead and change the type from ‘pie’ to ‘line’.
Line Graph
// The type of chart we want to createtype: 'line',
If we open our index.html we should see something like this.

Chart.js gives us multiple styles of charts like pie, line and many more.
Doughnut Graph
// The type of chart we want to createtype: 'doughnut',
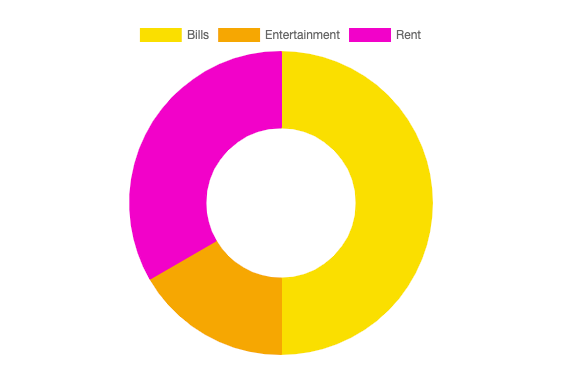
Radar Graph
// The type of chart we want to createtype: 'radar',

Bar Graph
// The type of chart we want to createtype: 'bar',

Horizontal Bar Graph
// The type of chart we want to createtype: 'horizontalBar',

Polar Area Graph
// The type of chart we want to createtype: 'polarArea',

Bubble Chart
// The type of chart we want to createtype: 'bubble',

You’re now one step closer to showing off your creative charts in your presentation.

If you want to tailor your graphs a little bit more I have linked the website that goes over every feature that Chart.js includes.